What is an API? How Does It Work, Types, and Use Cases
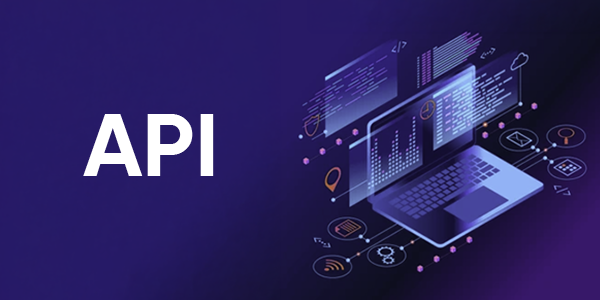
What is an API? What Does API Mean? Simple Definition and Importance
API (Application Programming Interface) serves as a bridge that enables different software to communicate with each other. It is a set of rules that allows one program to interact with another program.
For example, a mobile app needs to connect to a weather API to display weather data. This API allows the app to fetch data from a weather provider. While the user only sees the temperature and weather conditions on the app interface, the API retrieves and processes the data in the background.
The Importance of APIs
- Data and Service Sharing: Enables data flow between different platforms.
- Automation: Speeds up processes by reducing manual tasks.
- Easy Integration: Used to integrate third-party services into software.
- Scalability: Offers a flexible and efficient structure, even for large projects.
APIs are widely used in modern software, including web services, mobile apps, IoT devices, financial systems, and many other areas.
How Does an API Work? Step-by-Step Explanation
To understand how an API works, we need to examine the communication process between the client and the server. The API acts as a bridge between these two sides, managing data exchange.
API Work Process
The Client Sends a Request:
- The user wants to access specific data through an app or website.
- For example, a weather app sends a request to the API asking, "What is the current temperature?"
The API Processes the Request and Forwards It to the Server:
- The API receives the client's request, converts it into a readable format, and forwards it to the relevant server.
- At this stage, HTTP requests (GET, POST, PUT, DELETE) are commonly used.
The Server Processes the Request and Returns a Response:
- The server processes the incoming request and sends the appropriate response back to the API.
- In the weather app example, the server retrieves the current weather data and sends it to the API.
The API Receives the Response and Sends It to the Client:
- The API receives the response from the server, organizes it, and forwards it to the client.
- The user sees the temperature on the app interface.
Example of How an API Works:
To better understand the API's operation, let's consider an e-commerce platform.
- The user opens the "My Cart" page on a mobile app.
- The app sends a request to the API, asking, "What items are in this user's cart?"
- The API connects to the database, retrieves the items in the user's cart, and returns the data.
- The user views the items in the cart on the app.
This entire process happens in milliseconds, and although the user only sees an interface, the API is working intensively in the background.
What Does an API Do? Purposes and Benefits
APIs play a critical role in enabling data sharing and integration between different software, serving many different areas. Today, they have a wide range of applications, from mobile apps to large-scale enterprise systems.
Purposes of API Usage
The main purposes of APIs are:
Data Sharing and Access:
- APIs allow different systems to connect and exchange data.
- For example, a weather API allows multiple applications to access weather forecasts.
Integrating Third-Party Services:
- Through APIs, software can easily integrate external services.
- For example, e-commerce sites use payment systems (like PayPal, Stripe) via APIs.
Automation and Efficiency:
- APIs automate business processes by reducing manual tasks.
- For example, accounting software can automatically fetch bank account transactions using an API.
Scalability and Flexibility:
- APIs make it easy to scale systems, even in large projects.
- For example, an e-commerce platform can update stock information from various suppliers via an API.
Security and Authorization:
- APIs ensure security by allowing users to access only specific data.
- For example, a social media platform can grant third-party apps access to only certain data using systems like OAuth 2.0.
Benefits of APIs
- Easy Integration: Ensures seamless operation between different software.
- Faster Development: APIs speed up development by providing pre-built functionality, rather than coding new features from scratch.
- Cost Savings: Using existing APIs reduces development time and costs.
- Better User Experience: APIs help apps run faster and more smoothly, enhancing the user
How to Create an API? Development Process and Tips
Creating an API is done within a framework of specific rules and standards to ensure smooth communication between systems. During the API development process, important factors like design, security, and performance should be taken into consideration.
API Creation Process
Define the Purpose and Use Cases of the API
The first step in API development is to determine what problem it will solve and who will use it.
- What services will the API offer?
- What data format will be used (JSON, XML, etc.)?
- Who will have access to the API?
Example: If we want to develop an API for an e-commerce platform to list products, we need to determine how the API will work with other systems like order management and user authorization.
Choose the Type of API (REST, SOAP, GraphQL, etc.)
When creating an API, the architecture and protocol to be used should be selected.
- RESTful API: The most commonly used type of API. It works with the HTTP protocol and exchanges
SOAP API: It is XML-based and is particularly preferred in large enterprise systems.
GraphQL API: It offers flexible data querying and optimizes the retrieval of data based on the client's specific needs.
Suggestion: Most modern applications use RESTful APIs because they are lightweight and scalable.
Designing the API Request and Response Structure
The success of an API depends on having a consistent and understandable request/response system.
Example of a REST API Request:
To get the user list:
GET https://api.example.com/users
To create a new user:
POST https://api.example.com/users
Body: {
"name": "Kalm Works",
"email": "[email protected]"
}
To get the information of a specific user:
GET https://api.example.com/users/123
Here, HTTP methods are used:
- GET: To retrieve data
- POST: To add new data
- PUT/PATCH: To update existing data
- DELETE: To delete data
Taking Security Measures
APIs should be protected against unauthorized access.
- API Key: Used to authenticate users accessing the API.
- OAuth 2.0: Ensures secure user authentication.
- Rate Limiting: Prevents excessive API requests to avoid system overload.
- Data Encryption: Methods like SSL/TLS should be used to protect sensitive information.
Testing the API and Preparing Documentation
The API needs to be tested to ensure it works correctly and securely.
- Tools like Postman and Swagger are used to test API requests.
- Detailed documentation explaining how to access the API is created.
- Sample code is provided to help developers integrate the API easily.
How to Use an API? Integration Process and Applications
API usage allows software to communicate with external services, simplifying the development process. To use an API, it is necessary to know the request format, use the correct authentication methods, and be able to handle the incoming responses.
API Usage Process
Accessing the API
Before using an API, you need to obtain an access key (API Key) or OAuth authentication credentials from the API provider.
- Public API: Can be used by anyone. (e.g., weather APIs)
- Private API: Can only be used by specific users. (e.g., banking APIs)
- Authorized API: Requires user authentication. (e.g., Facebook or Google login APIs)
Sending an API Request
APIs typically work with HTTP requests (GET, POST, PUT, DELETE).
- GET: Retrieves data
- POST: Adds new data
- PUT/PATCH: Updates data
- DELETE: Deletes data
Example API Requests:
To get user information:
GET https://api.example.com/users/123
{
"id": 123,
"name": "Kalm Works",
"email": "[email protected]"
}
To add a new user:
POST https://api.example.com/users
Body: {
"name": "Kalm Works",
"email": "[email protected]"
}
To update a user's information:
PUT https://api.example.com/users/123
Body: {
"name": "Kalm Works",
"email": "[email protected]"
}
Processing API Responses
API responses are typically in JSON or XML format.
- When a successful response is returned, you will receive an HTTP 200 OK status code.
- In case of an error, error codes like HTTP 404 (Not Found) or 500 (Server Error) are returned.
For example, a JSON response from a weather API might look like this:
{
"city": "İstanbul",
"temperature": "15°C",
"status": "Bulutlu"
}
By using this response within an application, we can display the real-time weather information to the user.
What Does API Usage Offer to Developers?
- APIs make it easy to add additional features to applications.
- By utilizing third-party services, it accelerates the development process.
- It automates manual processes, saving time and costs.
What Are the Features of an API? Basic Structure and Standards
APIs have specific features to ensure efficient, secure, and scalable communication between software systems. For an API to be successful, it must be fast, secure, flexible, and well-documented.
1. Core Features of an API
Platform Independence
APIs can work with different programming languages and operating systems. For example, a REST API can be called by both Python and JavaScript-based applications.
Data Format Support (JSON, XML)
Most modern APIs use the JSON (JavaScript Object Notation) format for data exchange. However, some APIs may still support XML (Extensible Markup Language) format.
Security (Authentication and Authorization)
APIs use various security methods to prevent unauthorized access:
- API Key: Defines access rights for users to the API.
- OAuth 2.0: Commonly used for user authentication (e.g., Google or Facebook APIs).
- JWT (JSON Web Token): Uses a secure token containing user information.
Error Management and Feedback
APIs use HTTP status codes to provide feedback on requests:
- 200 OK → Successful request
- 400 Bad Request → Invalid request
- 401 Unauthorized → Authentication error
- 404 Not Found → Requested data not found
- 500 Internal Server Error → Server error
Rate Limiting (Request Limiting)
API providers limit the number of requests made within a certain time period to prevent abuse and overload. For example, an API may accept a maximum of 100 requests per second.
Well-Documented
The user guide of an API accelerates the integration process for developers. For example, tools like Swagger, Postman, or Redoc can be used to create API documentation.
2. API Standards and Protocols
APIs are developed according to specific standards and protocols.
REST (Representational State Transfer)
- The most widely used API type.
- Uses HTTP methods (GET, POST, PUT, DELETE).
- Exchanges data in JSON format.
SOAP (Simple Object Access Protocol)
- XML-based and secure.
- Used in large enterprise systems.
GraphQL
- Allows fetching multiple pieces of data in a single request.
- Particularly suitable for data-centric applications.
gRPC (Google Remote Procedure Call)
- Developed for high-speed and low-latency API calls.
- Preferred in large-scale microservice architectures.
3. Additional Features That Enhance API Quality
Versioning (API Versioning)
Ensures backward compatibility by creating different API versions. For example:
- https://api.example.com/v1/users
- https://api.example.com/v2/users
Caching
Improves performance by caching API responses.
Webhook Support
Allows the API to send notifications when certain events occur.
Why Are the Core Features of an API Important?
- For an API to be fast, secure, and flexible, the correct standards must be used.
- Well-structured APIs simplify software development processes.
- Documentation and error management ensure efficient use of the API.
What is a Web API? Differences and Use Cases
A Web API is a type of API that works over the internet, typically using the HTTP protocol, to facilitate data sharing and interaction. Web APIs allow communication between different systems and platforms through web-based services. These APIs are widely used for data exchange between applications and devices.
Key Features of a Web API
Works Over the HTTP Protocol
Web APIs operate over the internet's fundamental communication protocol, HTTP (Hypertext Transfer Protocol). Requests to the API are made using HTTP methods (GET, POST, PUT, DELETE).
Data Sharing in JSON or XML Format
Data exchange is typically done in JSON (JavaScript Object Notation) format, although some Web APIs also support XML. JSON is preferred by most Web APIs due to its human-readable and lightweight structure.
Platform and Language Independence
Web APIs can be accessed from different programming languages and operating systems. This provides flexibility for developers, as Web APIs are compatible with multiple platforms.
Differences of Web API
Web API vs. REST API
Web API is a broader term, while REST API is a subset of such APIs. A Web API can refer to any type of API that works over HTTP, whereas a REST API refers specifically to APIs that follow certain design principles. RESTful APIs are often Web APIs, but Web APIs are not necessarily RESTful.
Web API vs. SOAP API
SOAP APIs generally provide a heavier and more secure structure. Web APIs typically work with lighter protocols such as REST or JSON-RPC, making them faster and more flexible. SOAP is XML-based and tends to be more complex and less flexible.
Use Cases of Web API
The use cases of Web APIs are vast, and many modern applications are integrated with several Web APIs. Here are some common use cases:
E-Commerce and Payment Systems
E-commerce platforms use payment APIs (PayPal, Stripe) to allow users to make secure payments. Additionally, shipping tracking APIs notify customers about the status of their orders.
Map and Location-Based Services
Services like Google Maps API allow developers to integrate map, location data, and routing features into their applications. For example, Uber and Lyft use Web APIs to track user locations.
Social Media Integration
Social media platforms use Web APIs, such as Facebook, Twitter, and Instagram APIs, to add features like social media sharing and login to applications. Users can link their social media accounts to the application via these APIs.
Weather and Meteorological Data
Many weather apps pull data from services like OpenWeatherMap API. This data is displayed to users through mobile apps or websites.
Finance and Banking
Banks and financial institutions use Web APIs to offer services like account balance checking, money transfers, and currency exchange rate information.
Advantages of Web API
Multi-Platform Support
Web APIs can be used on both desktop and mobile devices, offering broad access for developers.
Flexibility
Web APIs can accept requests from various clients (mobile apps, web apps, IoT devices).
Scalability
Web APIs can be easily scaled to handle high traffic loads.
Time and Cost Savings
Instead of building in-app features from scratch, integrating third-party Web APIs is faster and more cost-effective.
Importance of Using Web APIs
Web APIs play a crucial role in facilitating data exchange between applications and integrating different systems. Using Web APIs saves time and makes applications stronger and more flexible.
What are the Use Cases of APIs?
APIs speed up processes and enable system integrations in many industries. Here are some common use cases:
E-Commerce and Retail
Payment APIs for secure transactions, shipping tracking, and inventory management.
Finance and Banking
Payment APIs, bank account management, and exchange rate APIs for financial transactions.
Healthcare
Electronic health records and telemedicine APIs for healthcare services management.
Education
Student information systems and educational material APIs for remote learning.
Travel and Tourism
Flight and hotel booking APIs, map APIs for travel planning.
Social Media
Social media APIs, messaging APIs for communication and content sharing.
APIs digitize and accelerate business processes in these sectors, while also simplifying integration.
API’s Advantages and Disadvantages
APIs offer numerous benefits but also come with some limitations. The table below summarizes the advantages and disadvantages of APIs:
Advantages | Disadvantages |
---|---|
Easy Integration: Facilitates data sharing between different systems. | Security Risks: APIs can be vulnerable to malicious attacks. |
Time-Saving: Ready-made APIs allow quick feature addition. | Dependency: Can create dependency on third-party API providers. |
Flexibility and Scalability: Offers flexibility for system expansion and adding new functionalities. | Low Performance: Data retrieved via APIs may sometimes be slow. |
Increased Efficiency: Speeds up business processes through automation and data sharing. | High Costs: Some API services can be expensive, especially with high traffic demands. |
API Examples and Use Cases
APIs are used across many popular platforms for various purposes. Here are some common API examples and their use cases:
- Google Maps API: Provides map and navigation services. It is used for location services and route creation.
- PayPal API: Used for payment processing on e-commerce websites. It allows websites to accept online payments from customers.
- Twitter API: Used for sending tweets, analyzing followers, and sharing content on the social media platform Twitter.
- OpenWeather API: Provides weather data. It offers real-time weather information.
- Stripe API: Commonly used for payment processing. It enables websites to easily accept credit card payments.
- Spotify API: Provides music streaming services. Users can stream music and create playlists.
APIs, like the examples above, simplify operations and integrate digital services across various industries.
Frequently Asked Questions About APIs
Are APIs free?
Many APIs offer free usage for limited access, but high-traffic or premium-feature APIs are generally paid. Paid APIs often provide additional features, faster response times, and better security.
How long does API integration take?
The time required for API integration depends on the complexity of the API and the integration process. Simple APIs can be integrated in a few hours, while more complex ones may take days or even weeks.
What programming languages do I need to learn to use an API?
There's no need to learn a specific programming language for using APIs. However, it helps to have basic knowledge of languages like HTML, JavaScript, Python, or PHP, as most APIs require sending and receiving HTTP requests.
How are API limits determined?
API providers typically set usage limits (rate limits) on a minute, hourly, or daily basis. These limits are designed to ensure the API operates smoothly and to prevent it from being overwhelmed by excessive requests.
Are APIs used only for web applications?
No, APIs can be used for more than just web applications. They are also used in mobile apps, desktop software, and even IoT devices.
How are API version updates handled?
API providers update their APIs with new versions, often to add features or address security issues. For the integration to work correctly, the application must support these updates as well.
Conclusion and Future Outlook on APIs
APIs (Application Programming Interfaces) play a critical role in modern software development. By enabling different systems and applications to interact with each other, they make data sharing and business processes in the digital world much more efficient. The advantages provided by APIs offer great potential, particularly in terms of speed, flexibility, and integration. More and more industries are adopting API-based solutions, accelerating digital transformation with these technologies.
Benefits of APIs:
- Fast and Efficient Integration: APIs enable quick integration between different software systems, which accelerates business processes and makes them more efficient.
- Customizable Solutions: APIs can be customized according to user needs. They can be designed for various purposes, such as payment processing or obtaining weather data.
- Increased Efficiency: APIs automate business processes and make data sharing faster, which allows businesses to operate more efficiently.
- Security and Data Privacy: APIs ensure secure data transmission with high-level security measures. API providers protect user data using strong encryption methods.
Future Possibilities and Technological Advancements:
APIs are expected to play an even more significant role in the future. With technologies such as artificial intelligence, the Internet of Things (IoT), and machine learning, APIs will continue to evolve. By accelerating communication between systems and applications, APIs will speed up the pace of digital transformation.
- Artificial Intelligence and APIs: APIs will become more powerful with artificial intelligence and machine learning technologies. In fields like natural language processing (NLP) and deep learning, solutions provided by APIs will enable more accurate and faster analyses.
- IoT and APIs: With more devices connecting through the Internet of Things, APIs will continue to play an important role. The ability for devices to send data to one another will be made possible through APIs, facilitating the creation of more efficient, smart systems.
In conclusion, APIs will continue to be a rapidly growing and evolving field in the tech world. The advantages they offer to both corporate and individual users make them indispensable in the digital world. In the future, APIs will provide stronger, faster, and more efficient solutions, simplifying the integration of digital systems and contributing to the growth of businesses.
To explore other blog posts, you can visit our blog page. To discover our portfolio and learn more about our services, feel free to check out our portfolio or contact us. If you have any questions, we at kalm.works are happy to assist you.